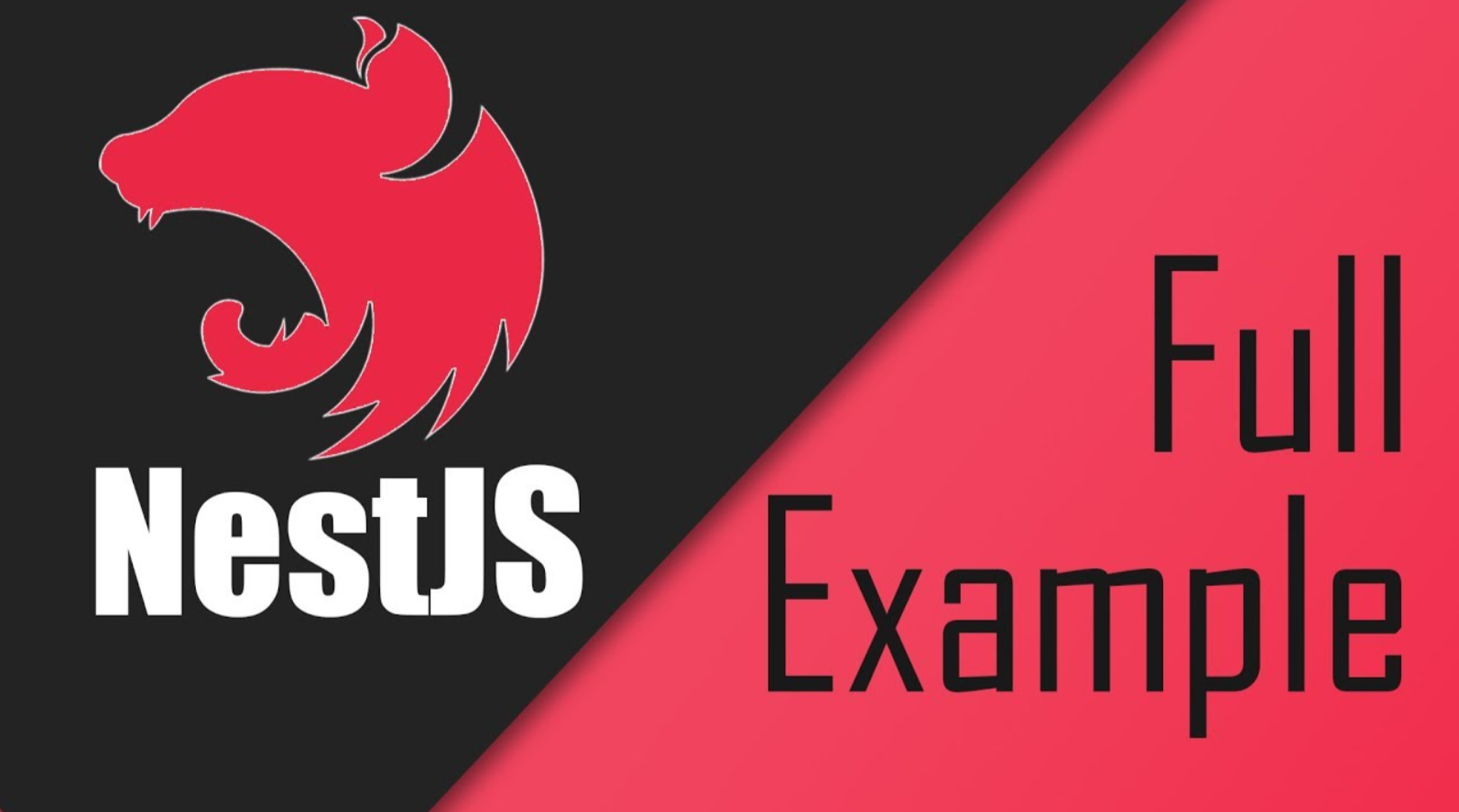
Khóa học Nestjs - Bài 02 - Giới thiệu mô hình MVC
Post Date : 2023-06-20T14:57:33+07:00
Modified Date : 2023-06-20T14:57:33+07:00
Category: nestjs-tutorial
Tags: nestjs , nestjs-pet-website
Source Code
Bài 02
- Phân tích yêu cầu thiết kế sơ bộ
- Thiết lập cấu trúc dự án
- Giới thiệu tổng quan về các lớp trong mô hình MVC và code thực hành
Phân tích yêu cầu
Sitemap
Functional details
Thiết kế cơ sở dữ liệu
Thiết lập cấu trúc dự án
- Tạo 1 dự án mới với NestJS
- Cấu trúc thư mục dự án theo cấu trúc module và mô hình MVC
- Debug dự án NestJS với VSCode
Tạo 1 dự án mới với NestJS
nest new nestjs-tutorial-2023 --skip-install
cd nestjs-tutorial-2023
npm i
Sau khi khởi tạo dự án , cấu trúc cây thư mục của dự án sẽ giống như hình bên dưới:
Cấu trúc thư mục dự án theo cấu trúc module và mô hình MVC
- Cài đặt NestJS hỗ trợ template Engine EJS
Chúng ta cùng quay lại một chút với kiến trúc của expressjs, bạn cần thiết lập 1 số thông số như sau:
- Template Engine : một số template engine phổ biến như pug, ejs, hbs
- Static Assets: thư mục chứa các file tĩnh như css, js, images của website
const express = require("express");
const app = express();
const port = process.env.PORT || 1337;
const path = require("path");
// static assets
const publicAssetsPath = path.resolve(process.cwd(), "public");
// using express static middleware
app.use("/public", express.static(publicAssetsPath));
// Your asset will be placed at : http://localhost:1337/public/assets/main.css
// select view engine is ejs
app.set("view engine", "ejs");
// set view folder
const viewsPath = process.cwd() + "/views";
app.set("views", [viewsPath]);
// global variable through app
app.locals = {
title: "MVC Tutorial",
};
// home page
app.get("/", (req, res) => {
res.render("index", { heading: "Home page" });
});
app.listen(port, () => {
console.log(`Example app listening at http://localhost:${port}`);
});
Còn trong NestJS, chúng ta sẽ làm thế nào
npm install --save @nestjs/serve-static
npm i --save ejs
// src/app.module.ts
import { Module } from "@nestjs/common";
import { AppController } from "./app.controller";
import { AppService } from "./app.service";
import { ServeStaticModule } from "@nestjs/serve-static";
import { join } from "path";
@Module({
imports: [
// public folder
ServeStaticModule.forRoot({
rootPath: join(process.cwd(), "public"),
// Your asset will be placed at : http://localhost:1337/public/assets/main.css
serveRoot: "/public",
}),
],
controllers: [AppController],
providers: [AppService],
})
export class AppModule {}
// src/main.ts
import { NestFactory } from "@nestjs/core";
import { AppModule } from "./app.module";
import { NestExpressApplication } from "@nestjs/platform-express";
import { join } from "path";
async function bootstrap() {
const app = await NestFactory.create<NestExpressApplication>(AppModule);
// app.useStaticAssets(join(process.cwd(), 'public'));
app.setViewEngine("ejs");
app.setBaseViewsDir([join(process.cwd(), "views")]);
await app.listen(3000);
}
bootstrap();
// src/app.controller.ts
import { Controller, Get, Render } from "@nestjs/common";
import { AppService } from "./app.service";
@Controller()
export class AppController {
constructor(private readonly appService: AppService) {}
@Get()
@Render("index")
homePage() {
return {};
}
}
views/index.ejs
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title><%= title %></title>
<link rel="stylesheet" href="/public/assets/main.css" />
</head>
<body>
<h1><%= title %></h1>
<img
src="/public/assets/nestjs-tutorial-2023.png"
alt="NestJS Tutorial 2023"
/>
<script src="/public/assets/main.js"></script>
</body>
</html>
And here is what you get